Pushing records from your EMR/EHR into OnCall Health in bulk
This guide is ideal for intermediate-level developers
To gain value from this guide, you'll need a decent understanding of JavaScript and REST APIs. Avoid copying and pasting code unless you understand how it works – especially when building an application that uses an OnCall Health API key, which grants access to sensitive patient data.
If you are an enterprise client and would like assistance, contact your Customer Success Manager.
If your healthcare organization is well-established, you likely have a substantial amount of data stored in an electronic medical record (EMR) or electronic health record (EHR) system. Pulling that information into OnCall Health as part of a one-time migration as your organization begins using our platform is a breeze thanks to our open API.
In this guide, we'll provide a high-level overview of two what it takes (in terms of API calls and architecture) to build an application that facilitates this migration.
Before you get started
Before proceeding, make sure you've met all of the requirements outlined on this page.
A note regarding endpoints
Throughout this guide, the endpoints we'll reference follow this format https://api.oncallhealth.ca/{uri}. If your healthcare organization is based in the United States, change .ca to .us for each endpoint. We have separate servers in either country to ensure HIPAA and PHIPA compliance.
Here are two options to consider when building the application that will facilitate this transfer of information.
Option 1: A full-stack application that parses the contents of a CSV file to create records in OnCall Health
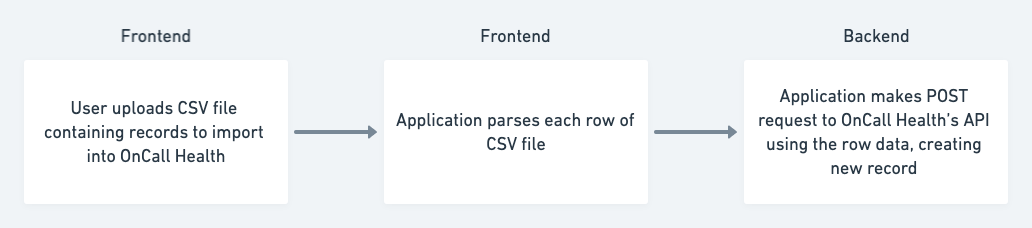
This option entails building a simple full-stack application (i.e. React.js frontend and Node.js backend) staff members within your healthcare organization can use to upload a CSV file containing records exported from your EMR or EHR. The application will parse that file and send its contents to OnCall Health's API.
This option makes sense if you would like non-technical staff members within your healthcare organization to be capable of creating bulk records in OnCall Health without your assistance.
Here's what the frontend and backend of your application would need to do.
Frontend
Step 1: Accept a CSV file containing one row for each record you'd like to create in OnCall Health
Each record's columns should contain values for the fields associated with its OnCall Health object type (see a full map of our API-accessible entities here).
For example, a CSV file containing patient records that need to be turned into roster contacts in OnCall Health should look something like this.
Name | Division | Provider | Sex | |
---|---|---|---|---|
John Smith | [email protected] | https://api.oncallhealth.ca/divisions/1/ | https://api.oncallhealth.ca/providers/1/ | male |
Step 2: Parse each row and send it to your application's backend
Next, your application should parse the CSV file and send its contents to the backend. An easy approach would be to do this row by row. Here's what parsing a row might look like.
// Inside the function that iterates through the CSV file
let data = {
name: row[0],
email: row[1],
division: row[2],
provider: row[3],
sex: row[4]
}
Note
For security and to avoid passing incorrect data into OnCall Health, consider implementing client-side validation. For example, you might want to implement logic that ensures the user input is a CSV file containing the expected headers. You may also want to check each row's individual columns (i.e. ensuring the "Name" column does not contain an email address, and so forth).
After parsing the data and storing it in an object (i.e. the hash demonstrated above), your application's frontend should send that data to the backend.
Backend
Step 3: Validate the data received from your application's frontend
OnCall Health's API is equipped to handle a variety of edge cases (including missing fields) but you may want to perform additional checks based on your organization's needs. Contact your Customer Success Representative if you're curious about particular edge cases.
Step 4: Form and make a POST request to the appropriate OnCall Health API endpoint
Using the above example, here's the POST request body your application's backend would need to form.
{
"name":"John Smith",
"provider":"https://api.oncallhealth.ca/providers/1/",
"division":"https://api.dev.oncallhealth.ca/divisions/1/",
"email":"[email protected]",
"sex":"male"
}
It would make this request to the https://api.oncallhealth.ca/contacts/ endpoint using your OnCall Health API key in the Authorization header.
Check out this guide for instructions on creating a Node.js service application that performs this function (see the /contacts endpoint in particular).
Note: Some OnCall Health entities have metadata fields that can be written to through the API. When applicable, we strongly recommend storing a reference to the corresponding EMR or EHR record in that metadata field. This will help you match the records later on.
Option 2: A service application that retrieves records directly from your EMR or EHR and creates records in OnCall Health using their data

This option entails building a server-side application that makes GET calls to your EMR or EHR's API and POST calls to OnCall Health's API. Here's what that application needs to do.
Step 1: Make a GET call to your EMR or EHR's API that retrieves all of the records you'd like to import into OnCall Health
If you're looking to transfer patient chart data into OnCall Health, for example, you'll need to make a GET call that retrieves all patient charts in your EMR or EHR.
Step 2: Iterate through the records you retrieved in Step 1 and do the following
For each record, use its data to form the body of a POST request to the appropriate OnCall Health API endpoint.
If you're looking to create appointment objects in OnCall Health, for example, you'll need the following (required) fields.
{
"title":"",
"datetime":"",
"duration":0,
"type":"",
"division":"",
"participants":[
{
"name":"",
"email":"",
"fee":
}
]
}
Note: Some OnCall Health objects (including appointments) have metadata fields. We strongly recommend storing a reference to the corresponding EMR or EHR record (i.e. the record's primary key) in those fields whenever possible. This will make reconciling records between OnCall Health and your EMR or EHR later on easy.
Of course, not every required field in OnCall Health will correspond with information available in your EMR or EHR. The division field in the above object, for example, requires a URL referencing the OnCall Health division in which you'd like to create an appointment. If you're an enterprise client, your Customer Success Manager can provide context regarding what values to use in fields you're uncertain about.
Next steps
After following the steps in this article, you should have the desired records from your EMR or EHR pulled into OnCall Health. Next, you may want to consider setting up automated transfers so you don't have to continually pull data into OnCall Health manually.
Here are some guides you may find useful for this purpose:
Updated over 3 years ago